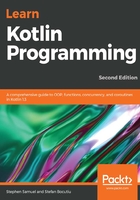
Member extension functions
Extension functions are usually declared at the top level, but we can define them inside classes as members. This may be used if we want to limit the scope of an extension:
class Mappings { private val map = hashMapOf<Int, String>() private fun String.stringAdd(): Unit { map.put(hashCode(), this) } fun add(str: String): Unit = str.stringAdd() }
In this example, we have defined an extension function that adds a string to hashmap. The second function just invokes this extension function. This roundabout way of adding to hashmap indicates how receivers work in member extension functions.
The hashCode function is defined on Any, and so it exists on the Mappings and String classes through inheritance. When hashCode is invoked in the extension function, there are two possible functions in scope that could be used. The first function in the Mappings instance is called the dispatch receiver. The second function on the string instance is called the extension receiver.
When we have this kind of name shadowing, the compiler defaults to the extension receiver. In the previous example, the hash code used will be the hash code of the string instance. To use the dispatch receiver, we must use a qualified this:
class Mappings { private val map = hashMapOf<Int, String>() private fun String.stringAdd(): Unit { map.put(this@Mappings.hashCode(), this) } fun add(str: String): Unit = str.stringAdd() }
In this second example, the hashCode function will be invoked on the Mappings instance.