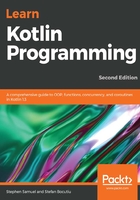
Companion object extensions
Extension functions can also be added to companion objects. They would then be invoked on the companion object rather than on instances of the class.
One example of where this might be useful is when you are adding factory functions to a type. For example, we might wish to add a function to integers in order to return a different random value upon each invocation:
fun Int.Companion.random(): Int { val random = Random() return random.nextInt() }
Then, we can invoke the extension function as normal, without needing the Companion keyword:
val int = Int.random()
This isn't as useful as regular extension functions. This is because we can always create a new object and put the function in there or create a top-level function. But you may wish to associate a function with some other type's namespace. As in the preceding example, a random() function invoked on the Int type is more intuitive than the same function on a class with a name such as IntFactory or RandomInts.