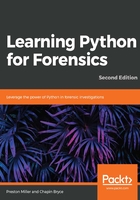
Using the raw input method and the system module – user_input.py
Both input() and sys.argv represent basic methods of obtaining input from users. Be cognizant of the fact that both of these methods return string objects, as previously discussed for the Python 2 raw_input() and Python 3 input() functions. We can simply convert the string into the required data type using the appropriate class constructor.
The input() function is similar to asking someone a question and waiting for their reply. During this time, the program's execution thread halts until a reply is received. We define a function later that queries the user for a number and returns the squared value. As seen in our first script, when converting Unix timestamps, we have to wait for the user to provide a value before the script can continue. While this wasn't an issue in that very short script, larger code bases or long-running scripts should avoid this delay.
Arguments supplied at the command line are stored in the sys.argv list. As with any list, these arguments can be accessed with an index, which starts at zero. The first element is the name of the script, while any element after that represents a space-separated user-supplied input. We need to import the sys module to access this list.
On line 39, we copy the arguments from the sys.argv list into a temporary list variable named args. This is preferred because, on line 41, we remove the first element after printing it. For the remaining items in the args list, we use a for loop and wrap our list with the built-in enumerate() function. This gives us a counter for our loop, i, to count the number of loop iterations or arguments used in this case. On lines 43 and 44, we print out each argument and its position and data type. We have the following code:
001 """Replicate user input in the console."""
002 from __future__ import print_function
003 import sys
...
033 __authors__ = ["Chapin Bryce", "Preston Miller"]
034 __date__ = 20181027
035 __description__ = "Replicate user input in the console"
036
037
038 def main():
039 args = sys.argv
040 print('Script:', args[0])
041 args.pop(0)
042 for i, argument in enumerate(sys.argv):
043 print('Argument {}: {}'.format(i, argument))
044 print('Type: {}'.format(type(argument)))
045
046 if __name__ == '__main__':
047 main()
After saving this file as user_input.py, we can call it at the command line and pass in our arguments.

For smaller programs that do not have many command-line options, the sys.argv list is a quick and easy way to obtain user input without blocking script execution.