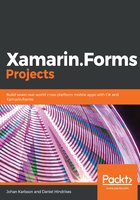
Creating a repository and its interface
Now that we have the TodoItem class, let's define an interface that describes a repository to store our to-do list items:
- In the .NET Standard library project, create a folder called Repositories.
- Create an interface called ITodoItemRepository.cs in the Repositories folder and write the following code:
using System;
using System.Collections.Generic;
using System.Threading.Tasks;
using DoToo.Models;
namespace DoToo.Repositories
{
public interface ITodoItemRepository
{
event EventHandler<TodoItem> OnItemAdded;
event EventHandler<TodoItem> OnItemUpdated;
Task<List<TodoItem>> GetItems();
Task AddItem(TodoItem item);
Task UpdateItem(TodoItem item);
Task AddOrUpdate(TodoItem item);
}
}
This interface defines everything we need for our app. It is there to create logical insulation between your implementation of a repository and the user of that repository. If any other part of your application wants an instance of TodoItemRepository, we can pass it any object that implements ITodoItemRepository, regardless of how it's implemented.
Having that said, let's implement ITodoItemRepository:
- Create a class called TodoItemRepository.cs.
- Enter the following code:
using DoToo.Models;
using System.Collections.Generic;
using System.IO;
using System.Threading.Tasks;
namespace DoToo.Repositories
{
public class TodoItemRepository : ITodoItemRepository
{
public event EventHandler<TodoItem> OnItemAdded;
public event EventHandler<TodoItem> OnItemUpdated;
public async Task<List<TodoItem>> GetItems()
{
}
public async Task AddItem(TodoItem item)
{
}
public async Task UpdateItem(TodoItem item)
{
}
public async Task AddOrUpdate(TodoItem item)
{
if (item.Id == 0)
{
await AddItem(item);
}
else
{
await UpdateItem(item);
}
}
}
}
This code is the bare-bones implementation of the interface, except for the AddOrUpdate(...) method. This handles a small piece of logic that states that if the ID of an item is 0, it's a new item. Any item with an ID larger than 0 is stored in the database. This is because the database assigns a value larger than zero when we create rows in a table.
There are also two events defined in the preceding code. These will be used for notifying any subscriber that items have been updated or added.