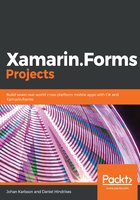
Creating a ValueConverter for the item status
Sometimes, we want to bind to objects that are a representation of the original value. This could be a piece of text that is based on a Boolean value. Instead of true and false, for example, we might want to write Yes and No, or return a color. This is where ValueConverter comes in handy. It can be used to convert a value to and from another value. We are going to write a ValueConverter that converts the status of a to-do list item to a color:
- In the root of the .NET Standard library project, create a folder called Converters.
- Create a class called StatusColorConverter.cs and add the following code:
using System;
using System.Globalization;
using Xamarin.Forms;
namespace DoToo.Converters
{
public class StatusColorConverter : IValueConverter
{
public object Convert(object value, Type targetType,
object parameter, CultureInfo
culture)
{
return (bool)value ?
(Color)Application.Current.Resources["CompletedColor"]:
(Color)Application.Current.Resources["ActiveColor"];
}
public object ConvertBack(object value, Type targetType,
object parameter, CultureInfo
culture)
{
return null;
}
}
}
A ValueConverter is a class that implements IValueConverter. This, in turn, only has two methods defined. The Convert method is called when the view reads data from the ViewModel, and the ConvertBack method is used when the ViewModel gets data from the view. The ConvertBack method is only used for controls that return data from plain text, such as the Entry control.
If we look at the implementation of the Convert method, we notice that any value passed into the method is of the object type. This is because we don't know what type the user has bound to the property to which we are adding this ValueConverter. We may also notice that we fetch colors from a resource file. We could have defined the colors in the code, but this is not recommended, so instead, we went the extra mile and added them as a global resource in the App.xaml file. Resources are a good thing to take another look at once we've finished with the chapter:
- Open App.xaml in the .NET Standard library project.
- Add the following ResourceDictionary:
<Application ...>
<Application.Resources>
<ResourceDictionary>
<Color x:Key="CompletedColor">#1C8859</Color>
<Color x:Key="ActiveColor">#D3D3D3</Color>
</ResourceDictionary>
</Application.Resources>
</Application>
A ResourceDictionary can define a wide range of different objects. We settle for the two colors that we want to access from the ValueConverter. Notice that these are accessible by the key given to them and they can also be accessed from any other XAML file using a static resource binding. The ValueConverter itself will be referenced as a static resource, but from a local scope.