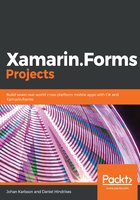
Navigating to an item using a command
We want to be able to see the details for a selected to-do list item. When we tap a row, we should navigate to the item in that row.
To do this, we need to add the following code:
- Open ViewModels/MainViewModel.cs.
- Add the SelectedItem property and the NavigateToItem method to the class:
public TodoItemViewModel SelectedItem
{
get { return null; }
set
{
Device.BeginInvokeOnMainThread(async () => await
NavigateToItem(value));
RaisePropertyChanged(nameof(SelectedItem));
}
}
private async Task NavigateToItem(TodoItemViewModel item)
{
if (item == null)
{
return;
}
var itemView = Resolver.Resolve<ItemView>();
var vm = itemView.BindingContext as ItemViewModel;
vm.Item = item.Item;
await Navigation.PushAsync(itemView);
}
The SelectedItem property is a property that we will data-bind to the ListView. When we select a row in the ListView, this property will be set to the TodoItemViewModel that represents that row. Since we can't really use Fody here to carry out its PropertyChanged magic, because of the need to do a method call in the setter, we need to go old-school and manually add a getter and a setter.
The setter then calls NavigateToItem, which creates a new ItemView using the Resolver. We extract the ViewModel from the newly created ItemView and assign the current TodoItem that the TodoItemViewModel contains. Confused? Remember that the TodoItemViewModel actually wraps a TodoItem and it is that item that we want to pass to the ItemView.
We are not done yet. We now need to data-bind the new SelectedItem property to the right place in the view:
- Open Views/MainView.xaml.
- Locate the ListView and add the attributes in bold:
<ListView x:Name="ItemsListView"
Grid.Row="1"
RowHeight="70"
ItemsSource="{Binding Items}"
SelectedItem="{Binding SelectedItem}">
The SelectedItem attribute binds the, SelectedItem property ListView to the ViewModel property. When the selection of an item in the ListView changes, the ViewModels SelectedItem property will be called and we will navigate to the new and exciting views.
The x:Name attribute is for naming the ListView, because we do need to make a small and ugly hack to make this work. The ListView will actually stay selected after the navigation is done. When we navigate back, it cannot be selected again until we select another row. To mitigate this, we need to hook up to the ItemSelected event of ListView and reset the selected item directly on the ListView. This is not recommended, because we shouldn't really have any logic in our Views, but sometimes we have no other choice:
- Open Views/MainView.xaml.cs.
- Add the following code in bold:
public MainView(MainViewModel viewmodel)
{
InitializeComponent();
viewmodel.Navigation = Navigation;
BindingContext = viewmodel;
ItemsListView.ItemSelected += (s, e) =>
ItemsListView.SelectedItem = null;
}
We should now be able to navigate to an item in the list.