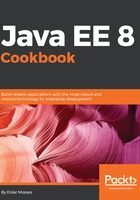
How it works...
The first thing to have a look at the following:
@ConcurrencyManagement(ConcurrencyManagementType.CONTAINER)
This is completely redundant! Singleton beans are container-managed by default, so you don't need to specify them.
Singletons are designed for concurrent access, so they are the perfect use case for this recipe.
Now let's check the LockType defined at the class level:
@Lock(LockType.READ)
@AccessTimeout(value = 10000)
public class UserClassLevelBean {
...
}
When we use the @Lock annotation at the class level, the informed LockType will be used for all class methods.
In this case, LockType.READ means that many clients can access a resource at the same time. It is usual for reading data.
In case of some kind of locking, LockType will use the @AccessTimeout annotation time defined to run into a timeout or not.
Now, let's check LockType defined at the method level:
@Lock(LockType.READ)
public int getUserCount(){
return userCount;
}
@Lock(LockType.WRITE)
public void addUser(){
userCount++;
}
So, here we are basically saying that getUserCount() can be accessed by many users at once (LockType.READ), but addUser() will be accessed just by one user at a time (LockType.WRITE).
The last case is the self-managed bean:
@ConcurrencyManagement(ConcurrencyManagementType.BEAN)
public class UserSelfManagedBean{
...
public synchronized void addUser(){
userCount++;
}
...
}
In this case, you have to manage all the concurrency issues for your bean in your code. We used a synchronized qualifier as an example.