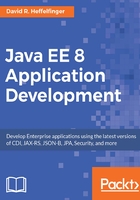
Navigation
As can be seen in our input page, when clicking on the Save button in the customer_data_entry.xhtml page, our application will navigate to a page called confirmation.xhtml. This happens because we are taking advantage of JSF's convention over configuration feature; if the value of the action attribute of a command button or link matches the base name of another page, then this navigation takes us to this page.
Same page reloading when clicking on a button or link that should navigate to another page? When JSF does not recognize the value of the action attribute of a command button or command link, it will by default navigate to the same page that was displayed in the browser when the user clicked on a button or link that is meant to navigate to another page.
If navigation does not seem to be working properly, chances are there is a typo in the value of this attribute. Remember that, by convention, JSF will look for a page whose base name matches the value of the action attribute of a command button or link.
The source for confirmation.xhtml looks like this:
<?xml version='1.0' encoding='UTF-8' ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://java.sun.com/jsf/html"> <h:head> <title>Customer Data Entered</title> </h:head> <h:body> <h:panelGrid columns="2" columnClasses="rightAlign,leftAlign"> <h:outputText value="First Name:"></h:outputText> <h:outputText value="#{customer.firstName}"></h:outputText> <h:outputText value="Last Name:"></h:outputText> <h:outputText value="#{customer.lastName}"></h:outputText> <h:outputText value="Email:"></h:outputText> <h:outputText value="#{customer.email}"></h:outputText> </h:panelGrid> </h:body> </html>
The <h:outputText> is the only tag on this page we haven't covered before. This tag simply displays the value of its value attribute to the rendered page, its value attribute can be a simple string or a value binding expression. Since the value binding expressions in our <h:outputText> tags are the same expressions used in the previous page for the <h:inputText> tags, their values will correspond to the data the user entered:
In traditional (that is, non-JSF) Java web applications, we define URL patterns to be processed by specific servlets. Specifically for JSF, the suffixes .jsf or .faces are commonly used; another commonly used URL mapping for JSF is the /faces prefix. Under certain conditions, modern application servers automatically add all three mappings to the faces servlet, if these conditions are met, we don't have to specify any URL mappings at all.
If any of these conditions are met, then the FacesServlet will be automatically mapped:
- There is a faces-config.xml file in the WEB-INF directory of our web application
- There is a faces-config.xml file in the META-INF directory of one of the dependencies of our web application
- There is a filename ending in .faces-config.xml in the META-INF directory of one of the dependencies of our web application
- We declare a context parameter named javax.faces.CONFIG_FILES in our web.xml or a web-fragment.xml in one of the dependencies
- We pass a non-empty set of classes when invoking the onStartup() method of ServletContextInitializer
When none of the preceding conditions are met, we need to explicitly map the Faces servlet in our web.xml deployment descriptor, as illustrated here:
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee http://xmlns.jcp.org/xml/ns/javaee/web-app_3_1.xsd" version="3.1"> <servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>javax.faces.webapp.FacesServlet</servlet-
class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>/faces/*</url-pattern>
</servlet-mapping> </web-app>
The URL we used for the pages in our application was the name of our Facelets pages, prefixed by /faces.