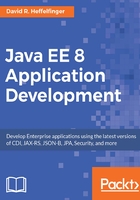
Creating custom validators
In addition to the standard validators, JSF allows us to create custom validators by creating a Java class implementing the javax.faces.validator.Validator interface.
The following class implements an email validator, which we will use to validate the email text input field in our customer data entry screen:
package net.ensode.glassfishbook.jsfcustomval; import javax.faces.application.FacesMessage; import javax.faces.component.UIComponent; import javax.faces.component.html.HtmlInputText; import javax.faces.context.FacesContext; import javax.faces.validator.FacesValidator; import javax.faces.validator.Validator; import javax.faces.validator.ValidatorException; import org.apache.commons.lang3.StringUtils; @FacesValidator(value = "emailValidator") public class EmailValidator implements Validator { @Override public void validate(FacesContext facesContext,
UIComponent uiComponent,
Object value) throws ValidatorException { org.apache.commons.validator.routines.EmailValidator emailValidator = org.apache.commons.validator.routines.EmailValidator.getInstance(); HtmlInputText htmlInputText = (HtmlInputText) uiComponent; String email = (String) value; if (!StringUtils.isEmpty(email)) { if (!emailValidator.isValid(email)) { FacesMessage facesMessage = new FacesMessage(htmlInputText. getLabel() + ": email format is not valid"); throw new ValidatorException(facesMessage); } } } }
The @FacesValidator annotation registers our class as a JSF custom validator class. The value of its value attribute is the logical name that JSF pages can use to refer to it.
As can be seen in the example, the only method we need to implement when implementing the Validator interface is a method called validate(). This method takes three parameters—an instance of javax.faces.context.FacesContext, an instance of javax.faces.component.UIComponent, and an object. Typically, application developers only need to be concerned with the last two. The second parameter is the component whose data we are validating, the third parameter is the actual value. In the example, we cast uiComponent to javax.faces.component.html.HtmlInputText; this way, we get access to its getLabel() method, which we can use as part of the error message.
If the entered value is not an invalid email address format, a new instance of javax.faces.application.FacesMessage is created, passing the error message to be displayed in the browser as its constructor parameter. We then throw a new javax.faces.validator.ValidatorException. The error message is then displayed in the browser; it gets there behind the scenes via the JSF API.
Apache Commons Validator: Our custom JSF validator uses Apache Commons Validator to do the actual validation. This library includes many common validations such as dates, credit card numbers, ISBN numbers, and emails. When implementing a custom validator, it's worth investigating whether this library already has a validator that we can use.
In order to use our validator on our page, we need to use the <f:validator> JSF tag. The following Facelets page is a modified version of the customer data entry screen. This version uses the <f:validator> tag to validate email:
<?xml version='1.0' encoding='UTF-8' ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml" xmlns:h="http://java.sun.com/jsf/html" xmlns:f="http://java.sun.com/jsf/core"> <h:head> <title>Enter Customer Data</title> </h:head> <h:body> <h:outputStylesheet library="css" name="styles.css"/> <h:form> <h:messages></h:messages> <h:panelGrid columns="2" columnClasses="rightAlign,leftAlign"> <h:outputText value="First Name:"> </h:outputText> <h:inputText label="First Name" value="#{customer.firstName}" required="true"> <f:validateLength minimum="2" maximum="30"> </f:validateLength> </h:inputText> <h:outputText value="Last Name:"></h:outputText> <h:inputText label="Last Name" value="#{customer.lastName}" required="true"> <f:validateLength minimum="2" maximum="30"> </f:validateLength> </h:inputText> <h:outputText value="Email:"> </h:outputText> <h:inputText label="Email" value="#{customer.email}">
<f:validator validatorId="emailValidator" />
</h:inputText> <h:panelGroup></h:panelGroup> <h:commandButton action="confirmation" value="Save"> </h:commandButton> </h:panelGrid> </h:form> </h:body> </html>
After writing our custom validator and modifying our page to take advantage of it, we can see our validator in action: