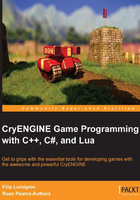
Creating a custom node in C#
CryMono also supports the creation of custom nodes using idioms that C# developers will feel accustomed to, such as attribute metaprogramming. To get started with C# CryENGINE scripts, open up the sample scripts solution in Game/Scripts/CryGameCode.sln
. Add a new .cs
file to the flownodes folder, and we'll start creating the same node in C#, so you can see how the creation varies.
To start with, let's create a basic skeleton node. We need to bring the correct namespace into scope for the Flowgraph
classes, as well as set up some basic attributes for our node:
using CryEngine.Flowgraph; namespace CryGameCode.FlowNodes { [FlowNode(Name = "Multiplier", Category = "CSharpTutorial", Filter = FlowNodeFilter.Approved)] public class TutorialNode : FlowNode { } }
As in C++, nodes aren't referenced anywhere else in the project, so we assign a separate namespace for our nodes to keep them from polluting the main namespaces.
We use the FlowNodeAttribute
class in place of GetConfiguration
to set up metadata for our node, such as the correct category and visibility level. Your node must include this attribute and inherit it from FlowNode
in order to be registered by the CryENGINE; there's no need for any manual registration calls.
Adding inputs
Inputs are defined as functions in CryMono, and they take either a single parameter which defines the data type, or no parameter for void ports. They also need to be decorated with the Port
attribute. In our case, let's set up the same three inputs we had in the C++ version of the node:
[Port] public void Activate() { } [Port] public void Left(float value) { } [Port] public void Right(float value) { }
We'll come back to the implementation of Activate
in just a second. While you can override the port name by setting optional parameters in the attribute, it's easier way to just let your function name define how the node appears in the Editor.
Adding outputs
Outputs are stored as instances of either OutputPort
or OutputPort<T>
, if values are required. Let's add our Result
output now as a property on the class:
public OutputPort<float> Result { get; set; }
Implementing Activate
Let's jump back to our Activate
input; again, we need to retrieve our two values and then fire an output. The FlowNode
class has convenient functions for these:
var left = GetPortValue<float>(Left); var right = GetPortValue<float>(Right); var answer = left * right; Result.Activate(answer);
That's it! Next time you open the Flowgraph Editor, you'll see your new CSharpTutorial:Multiplier node, with the exact same functionality as the C++ equivalent you implemented earlier:

Congratulations once again, as you've taken your first step to writing game code using the .NET platform and CryENGINE!
Target entities
Adding support for target entities in CryMono is easy, simply set the TargetsEntity
property in your FlowNode
attribute to true:
[FlowNode(TargetsEntity = true)]
You can then obtain the entity instance via FlowNode.TargetEntity
, assuming it was assigned inside the flowgraph containing the node.